Understanding Matplotlib in Python: A Comprehensive Guide to Plotting
- offpagework1datatr
- Apr 1, 2024
- 2 min read
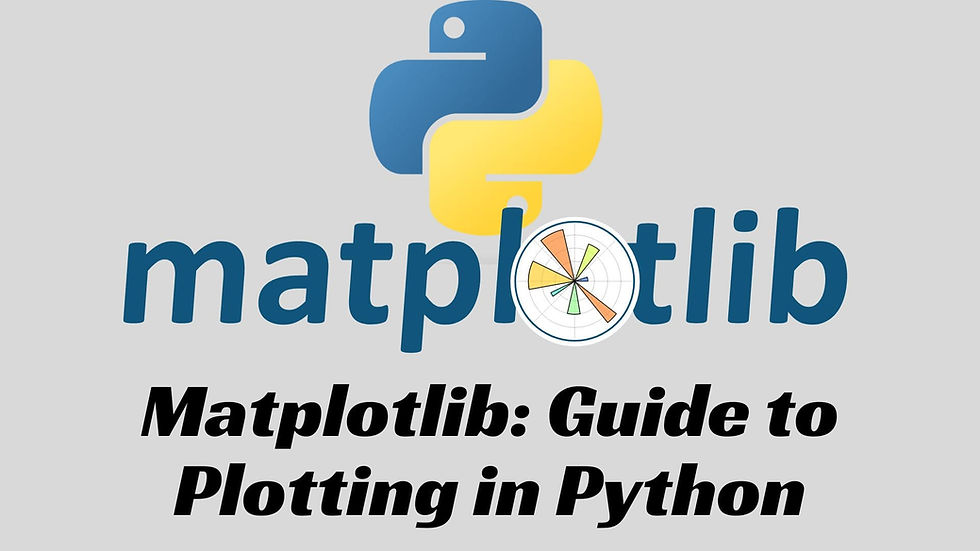
Matplotlib stands as one of the most widely used libraries for data visualization in Python. Whether you're a data scientist, a researcher, or a hobbyist programmer, Matplotlib offers a plethora of tools for creating high-quality plots and graphs.
What Matplotlib is, its features, and how you can leverage it effectively for plotting data in Python.
What is Matplotlib?
Matplotlib is a powerful plotting library for Python that enables users to create various types of visualizations, including line plots, scatter plots, bar plots, histograms, and more. Developed by John D. Hunter in 2003, Matplotlib was designed to replicate MATLAB's plotting capabilities in Python and has since become a cornerstone tool in the Python data science ecosystem.
Key Features of Matplotlib:
1. Simple Interface: Matplotlib provides a straightforward API for creating plots, making it accessible to users of all skill levels.
2. Extensibility: Users can customize virtually every aspect of their plots, from colors and line styles to axis labels and annotations.
3. Multiple Output Formats: Matplotlib supports a wide range of output formats, including PNG, PDF, SVG, and more, allowing users to save their plots in various file types.
4. Integration with Jupyter Notebooks: Matplotlib seamlessly integrates with Jupyter Notebooks, enabling interactive plotting within the notebook environment.
5. Wide Range of Plot Types: Whether you need to visualize numerical data, categorical data, or statistical distributions, Matplotlib offers a diverse array of plot types to suit your needs.
Getting Started with Matplotlib:
To begin using Matplotlib, you first need to install it using pip, Python's package manager:
```bash
pip install matplotlib
```
Once installed, you can import Matplotlib into your Python script or Jupyter Notebook using the following import statement:
```python
import matplotlib.pyplot as plt
```
Basic Plotting with Matplotlib:
Let's start by creating a simple line plot using Matplotlib. Suppose we have a set of x and y coordinates that we want to plot:
```python
import matplotlib.pyplot as plt
# Data
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# Create a line plot
plt.plot(x, y)
# Add labels and title
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Simple Line Plot')
# Display the plot
plt.show()
```
In this example, we first import Matplotlib, define our data points, create a line plot using `plt.plot()`, add labels and a title to the plot using `plt.xlabel()`, `plt.ylabel()`, and `plt.title()`, respectively, and finally display the plot using `plt.show()`.
Customizing Plots:
One of Matplotlib's strengths lies in its ability to customize plots to meet specific requirements. Let's explore some common customization options:
1. Changing Line Colors and Styles:
```python
plt.plot(x, y, color='red', linestyle='--', marker='o', markersize=8)
```
2. Adding Legends:
```python
plt.plot(x, y, label='Data')
plt.legend()
```
3. Changing Axis Limits:
```python
plt.xlim(0, 6)
plt.ylim(0, 12)
```
4. Adding Annotations:
```python
plt.text(3, 8, 'Max', fontsize=12)
```
These are just a few examples of the many customization options available in Matplotlib.
Conclusion:
Matplotlib is an indispensable tool for data visualization in Python. Its simplicity, versatility, and extensive customization options make it the go-to choose for generating plots and graphs across various domains. Whether you're visualizing data for analysis, presentations, or publications, Matplotlib empowers you to create compelling visualizations with ease.
Comments