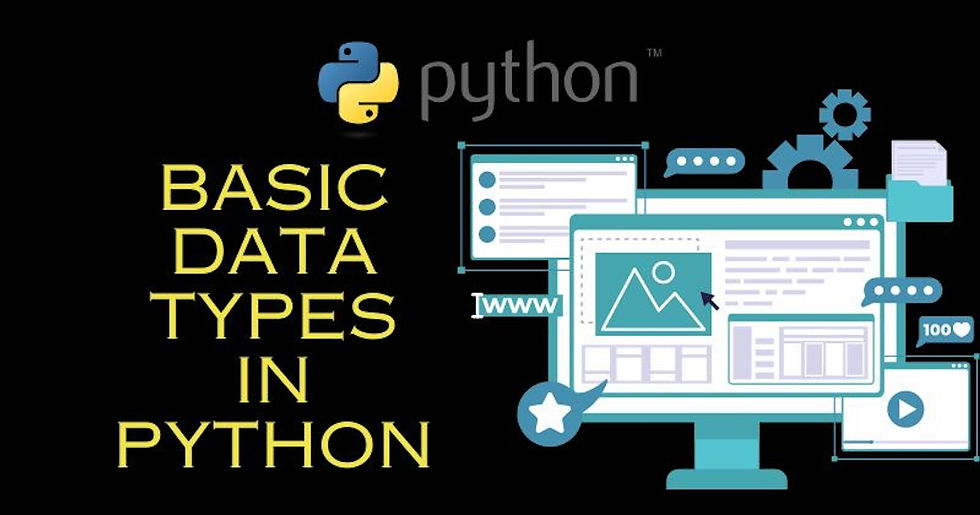
Python, being a dynamically typed language, offers a rich set of data types that cater to various programming needs. Understanding these data types is fundamental for writing efficient and readable code.
The basic data types in Python, exploring their characteristics, use cases, and operations.
1. Numeric Data Types:
Python supports several numeric data types, including integers, floating-point numbers, and complex numbers.
- Integers: Integers represent whole numbers without any decimal point. They can be positive, negative, or zero.
- Floating-point Numbers: Floating-point numbers, or floats, represent real numbers with a decimal point. They are used to represent values that require precision, such as scientific calculations or financial data.
- Complex Numbers: Complex numbers consist of a real part and an imaginary part (expressed as "x + yj"), where 'x' is the real part and 'y' is the imaginary part. They are useful in mathematical computations involving complex operations.
2. Sequence Data Types:
Sequence data types in Python represent ordered collections of items. The primary sequence data types are lists, tuples, and strings.
- Lists: Lists are mutable sequences that can contain elements of different data types. They are defined using square brackets [] and support various operations such as indexing, slicing, appending, and concatenation.
- Tuples: Tuples are immutable sequences similar to lists, but once created, their elements cannot be changed. They are defined using parentheses () and are commonly used to represent fixed collections of items.
- Strings: Strings represent sequences of characters and are immutable, meaning their contents cannot be altered after creation. They are defined using single quotes ('') or double quotes ("") and support numerous string manipulation operations.
3. Mapping Data Types:
Mapping data types in Python represent collections of key-value pairs. The primary mapping data type is the dictionary.
- Dictionaries: Dictionaries are mutable collections that store key-value pairs. They are defined using curly braces {} and are useful for storing and retrieving data efficiently based on keys. Dictionaries support operations such as key-value assignment, deletion, and iteration.
4. Set Data Types:
Set data types in Python represent unordered collections of unique elements. The primary set data type is the set.
- Sets: Sets are mutable collections that contain unique elements with no duplicate values. They are defined using curly braces {} or the set() constructor and support set operations such as union, intersection, difference, and symmetric difference.
5. Boolean Data Type:
The boolean data type in Python represents truth values, indicating either True or False.
- Boolean: Booleans are used to perform logical operations and control the flow of programs. They are often the result of comparison and logical operations and are essential for decision-making in programs.
Understanding the basic data types in Python is essential for writing efficient and robust code. By mastering these data types, developers gain the ability to manipulate data effectively, implement algorithms, and build complex software systems. Whether working with numbers, sequences, mappings, sets, or boolean values, Python provides a versatile set of tools to handle diverse programming tasks. With this comprehensive guide, you're equipped to leverage Python's data types to their fullest potential in your projects.
Comments